📦 Express.js & Socket.io Boilerplate
Simple Express.js & Socket.io Boilerplate.
✨ Features
- Express 4.16
- Mongoose 5.7
- Passport.js with Bcrypt.js
- Login endpoint
- Register endpoint
- Username availability check endpoint
- Authenticated endpoint
- Logout endpoint
- Socket.io integration for real-time, bidirectional and event-based communication.
- Generates automatically API docs based on Express existing routes with Swagger UI.
- Social login / register integration.
📝 Getting Started
git clone https://github.com/aaron5670/ExpressJS-SocketIO-Boilerplate.git
cd expressjs-socketio-boilerplate
npm install
// Optional: if you want dummy data, then run this seed file
node seed.js
node server.js
⚡ Create Socket.io connection
Install on your client-side (web) application the module socket.io-client.
npm install socket.io-client
Then create a connection with the following code:
// with ES6 import
import io from 'socket.io-client';
const socket = io('http://localhost:3005');
For more info you can read the official Socket.io documentation:
📓 How to document the Swagger UI endpoint API
/**
* @typedef ResponseJSON
* @property {string} username - user's username - eg: janet
* @property {string} message - message - eg: This is a authenticated route!
*/
/**
* Dashboard endpoint only allowed for authenticated users
* @route GET /api/v1/auth/dashboard
* @group Auth
* @returns {ResponseJSON.model} 200
* @produces application/json
*/
router.get('/dashboard', authenticationMiddleware(), (req, res) => {
return res.json({
username: req.session.passport.user.username,
message: 'This is a authenticated route!'
});
});
If you are running this boilerplate on you localhost you can see the Swagger UI documentation on: http://localhost:3005/api-docs/.
Note: Check if your port number is the same as your configuration.
📌 Swagger UI example
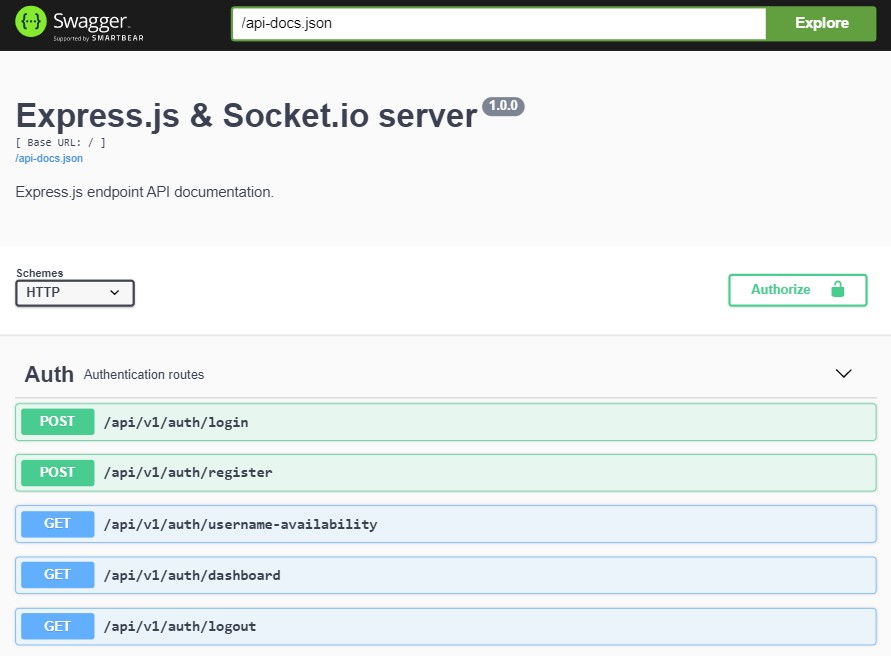
🚀 Endpoints
The following endpoints are available
Login
url: /api/v1/auth/login
method: POST
This request expects the following body:
{
"username": "jane",
"password": "securepassword1"
}
It will response the client the following object after a successful login:
{
"success": true,
"username": "jane",
"message": "Successful login, welcome!"
}
Register
url: /api/v1/auth/register
method: POST
This request expects the following body:
{
"name": "John Doe",
"username": "john",
"password": "password123"
}
It will response the client the following object after a successful register:
{
"success": true,
"message": "User is successfully registered!"
}
Username availability check
url: /api/v1/auth/username-availability
method: GET
query parameter: username
This request expects a request like:
https://localhost:3005/api/v1/auth/username-availability?username=john
It will response the client the following object after a successful request:
{
"usernameAlreadyInUsage": true
}
Logout
url: /api/v1/auth/logout
method: GET
It will response the client the following object after a successful request:
{
"success": true
}
Project details 49 18
View on GitHub